Sign In With Passkey Autofill
This guide extends Sign In With Passkey, allowing the user to authenticate using passkey autofill.
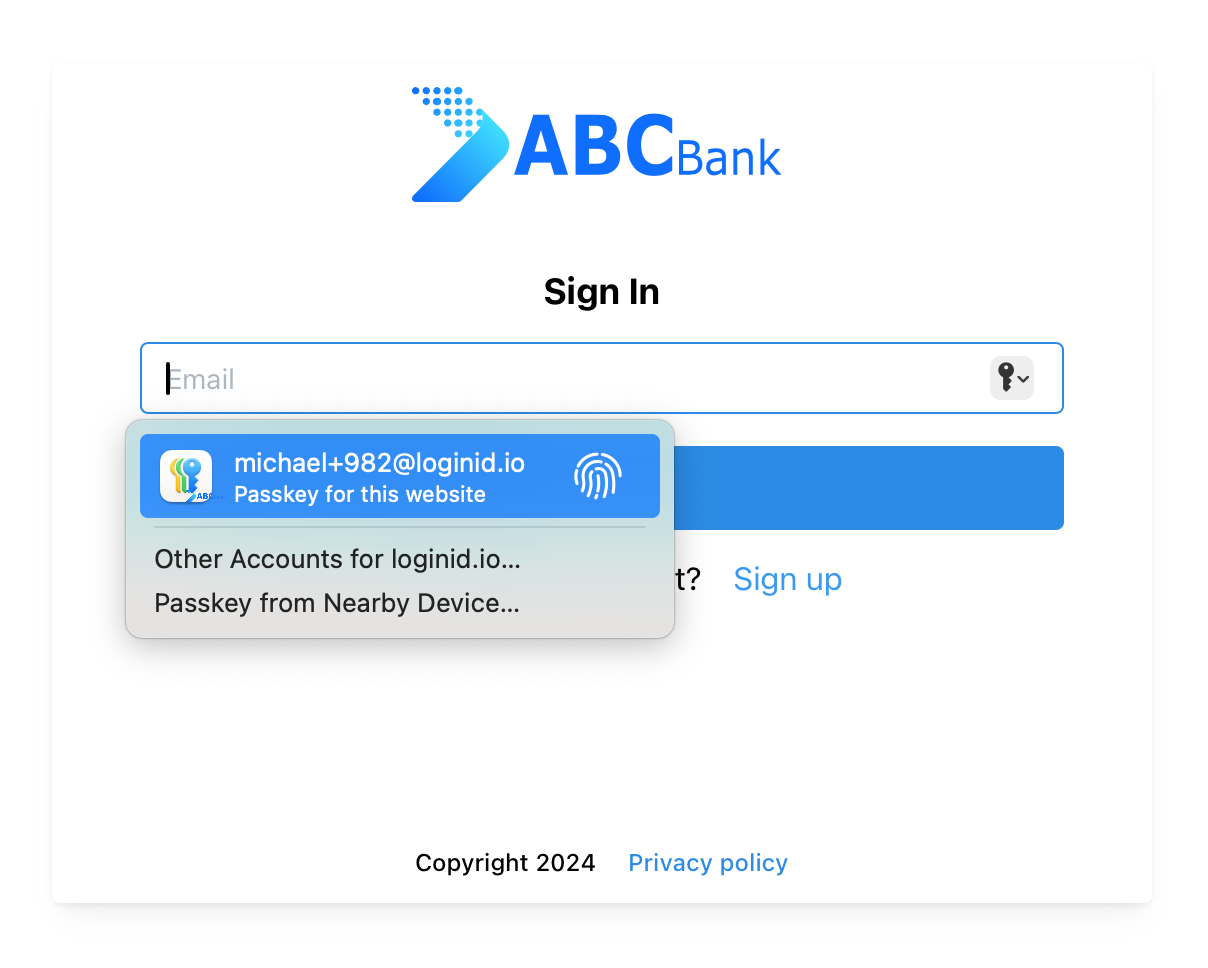
Prerequisites
- Create an application to obtain a base URL
Setup SDK
- Javascript
npm i @loginid/websdk3
Import and initialize an instance:
import { LoginIDWebSDK } from "@loginid/websdk3";
const lid = new LoginIDWebSDK({
baseUrl: process.env.LOGINID_BASE_URL,
});
Initialize a singleton configuration for the LoginID service in the application's onCreate
method.
class MyApp : Application() {
override fun onCreate() {
super.onCreate()
// Initialize the singleton instance
val config = LoginIDServiceConfig("<BASE_URL>")
LoginID.configure(config).client()
}
}
Configure the singleton instance of LIDClient
with a base URL during the app's launch in the AppDelegate
.
import LoginID
import UIKit
@main
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_: UIApplication, didFinishLaunchingWithOptions _: [UIApplication.LaunchOptionsKey: Any]?) -> Bool
{
// Initialize the singleton instance
LIDClient.shared.configure(baseURL: "<BASE_URL>")
return true
}
}
Passkey Autofill Example
- Javascript
Make sure to include the autocomplete attribute to the username input field with the value "username webauthn".
<form>
<label for="username">Email:</label>
<input
type="email"
id="username"
name="username"
autocomplete="username webauthn"
required
/>
<button type="submit">Submit</button>
</form>
To initiate passkey autofill call authenticateWithPasskeyAutofill during component or page load.
Here’s an example of how this looks in React using the useEffect hook.
import { LoginIDWebSDK } from "@loginid/websdk3";
// Initialize the SDK with your configuration
const config = {
baseUrl: process.env.BASE_URL,
};
const lid = new LoginIDWebSDK(config);
/*
* Setting up conditional UI and passkey login
*/
const Login: React.FC = () => {
const [username, setUsername] = useState<string>("");
const [error, setError] = useState<string>("");
// Start autofill
useEffect(() => {
const conditionalUI = async () => {
try {
const { token } = await lid.authenticateWithPasskeyAutofill();
// Submit token to the server.
} catch (e) {
if (e instanceof Error) {
setError(e.message);
}
}
};
conditionalUI();
}, []);
const handleSubmit = async (e: React.FormEvent) => {
e.preventDefault();
try {
const authResult = await lid.authenticateWithPasskey(username);
if (authResult.isAuthenticated) {
// You can return LoginID token to your backend for verification (authResult.token)
setAuthUser(user);
} else {
// Default to your fallback CIAM authentication method
}
} catch (e) {
if (e instanceof Error) {
setError(e.message);
}
}
};
return (
// This is a mini sample UI form
<form onSubmit={handleSubmit}>
<div>
<label htmlFor="username">Email:</label>
<input
type="email"
id="username"
name="username"
value={username}
onChange={(e) => setUsername(e.target.value)}
required
autocomplete="username webauthn"
/>
<button>Sign In</button>
</div>
</form>
);
};
Once you have received the result LoginID token, you can send it to your backend, and verify it. For detailed technical instructions, refer to this section on verifying LoginID tokens.