iOS
Initial Setup
The LoginID iOS SDK enables you to add passkey authentication in your native iOS application without having to redirect the user to any pages outside your application.
The SDK leverages the Authentication Services framework for creating and syncing passkeys with iCloud Keychain.
Required settings:
- Configure
apple-app-site-association
file - Base URL
The LoginID iOS Mobile SDK requires iOS 15+ for compatibility.
Configure apple-app-site-association File
In order for passkeys to work with your iOS application, you need to link your application with a website. You do this by creating an apple-app-site-association
and hosting it on your domain website. More info here.
You Need an Apple Developer Account
An Apple Developer Account is a requirement as the Associated Domains capability is not available for free. Currently, an Apple Developer Account can be obtained from https://developer.apple.com/support/enrollment.
Obtain Team ID and Bundler ID
- The team ID can be obtained on your developer account console at https://developer.apple.com/account.
- The bundler ID can be obtained on your
Signing & Capabilities
section of your application.
Host apple-app-site-association
JSON File on Your Website Directory
To host an apple-app-site-association
file, you need to serve it as a static JSON
file on your website. Note that the file must be named exactly apple-app-site-association
without the .json
extension and your server must be configured to serve it as JSON
. The file should be located at <WEBSITE_DOMAIN>/.well-known/apple-app-site-association
in the root directory of your website.
Here is an example of the minimum required fields in the file:
{
"webcredentials": {
"apps": ["<TEAM_ID>.<BUNDLER_ID>"]
}
}
Use the following example as a template and replace TEAM_ID
and BUNDLER_ID
with your values.
More information here.
Create an Associated Domains Capability on Your iOS App
To enable this capability
- You must have an Apple Developer Account
- Go to the
Signing & Capabilities
section of your application - Add a capability by clicking the
+ Capability
button - Choose
Associated Domains
- Enter the domain of your hosted website. Make sure to prefix it with
webcredentials
. Here's an example of what it should look like:
webcredentials:example.com
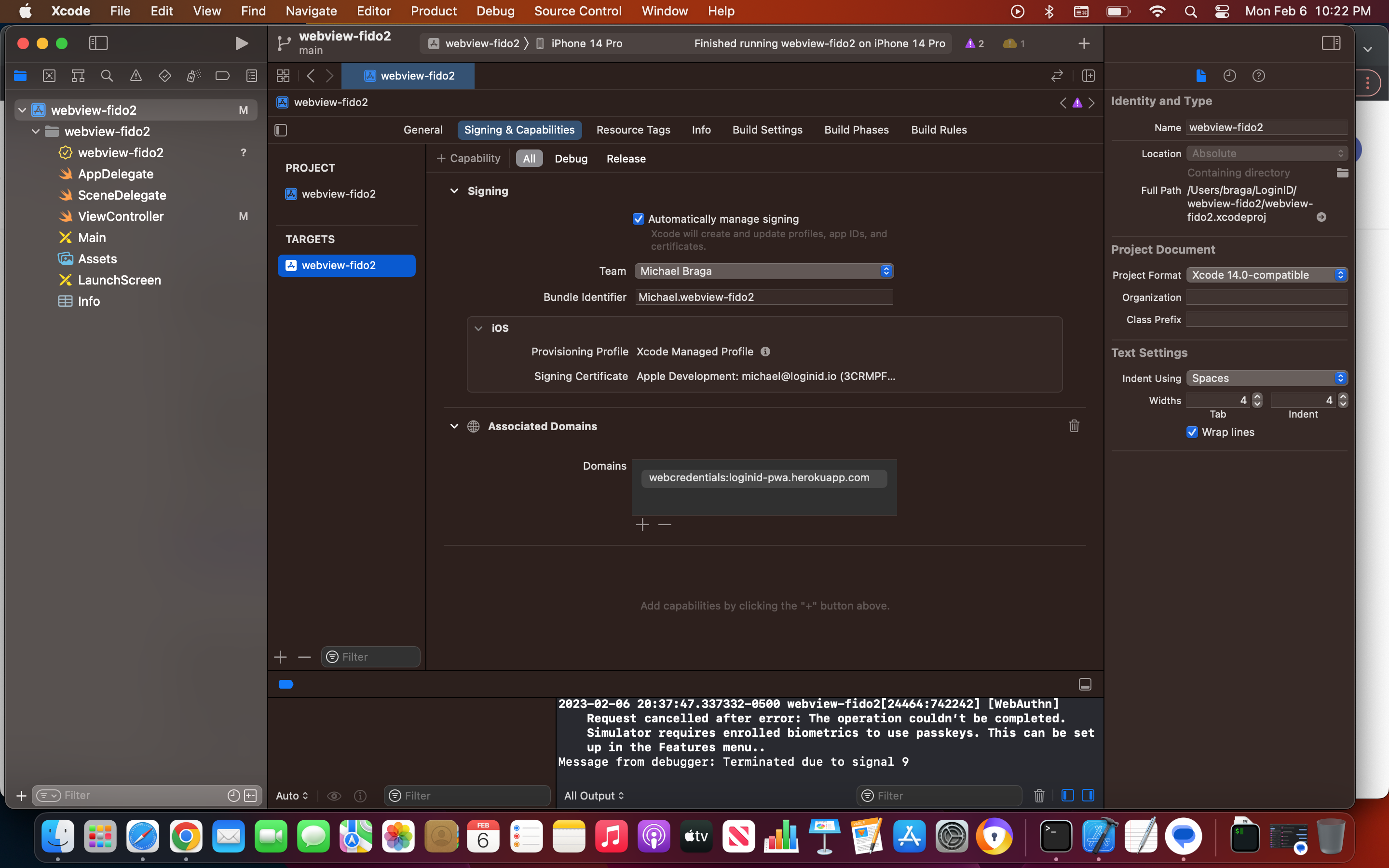
Create Application
Create a new application to obtain your base URL
.
Add SDK to Existing Application
Adding the SDK to your application currently requires a download and manual import via an .xcframework
.
Download the SDK
After creating an application, visit the iOS section in the Get Started
guide to download the latest SDK. The SDK is provided as an .xcframework
file, which will be imported into your application.
Import the Framework
Open your project in Xcode, then drag and drop the .xcframework
file into the Xcode Navigator area. Alternatively, go to General -> Frameworks, Libraries, and Embedded Content
to manually add it. Ensure it's set to Embed & Sign
. The SDK doesn't require any other external dependencies, making it simple to integrate.
Create an SDK Instance
The LoginID API must be called before any other APIs. You should call this API within your AppDelegate's didFinishLaunchingWithOptions
method.
- Swift
- Objective-C
import UIKit
import LoginIDSDK
...
@main
class AppDelegate: UIResponder, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
let baseURL = "<BASE_URL>"
LIDClient.shared.configure(baseURL: baseURL)
// Other setup code...
return true
}
...
...
#import <UIKit/UIKit.h>
#import "AppDelegate.h"
#import "LoginIDSDK/LoginIDSDK.h"
@interface AppDelegate ()
@end
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
NSString *baseURL = @"<BASE_URL>";
[[LIDClient shared] configureWithBaseURL:baseURL];
// Other setup code...
return YES;
}
...
API Reference
registerWithPasskey
Allows a user to sign up or add a new passkey using their username.
If options.usernameType
is set to email
(the default setting), the user will have a verified email profile.
Requires one of the following to add a new passkey to an existing user:
- User must be signed in.
- Usage of authorization tokens.
public func registerWithPasskey(
activity: ASPresentationAnchor,
username: String,
options: LIDOptions?,
onComplete: @escaping (LIDAuthResponse?, LIDApiError?) -> Void
)
Parameter | Type | Required | Details |
---|---|---|---|
anchor | ASPresentationAnchor | Yes | A presentation anchor, typically a window or a view controller, that the registration UI should be presented from. |
username | String | Yes | The username for the account being registered. |
options | LIDOptions | No | Additional options for the sign-up process. |
options.displayName | String | No | A human-palatable name for the user account, intended only for display on your passkeys and modals. |
options.usernameType | String | No | Specify username type validation. Types include email or phone . |
options.token | String | No | The user's authentication token, which may also be a management token with the scope passkey:write . |
onComplete | Closure | Yes | A completion handler that is called when the registration process completes, returning either an LIDAuthResponse or an LIDApiError . |
authenticateWithPasskey
Sign in a previously registered user with a passkey.
public func authenticateWithPasskey(
activity: ASPresentationAnchor,
username: String,
options: LIDOptions?,
onComplete: @escaping (LIDAuthResponse?, LIDApiError?) -> Void
)
Parameter | Type | Required | Details |
---|---|---|---|
anchor | ASPresentationAnchor | Yes | A presentation anchor, typically a window or a view controller, that the authentication UI should be presented from. |
username | String | Yes | The username for the account attempting to authenticate. Passing an empty string will trigger usernameless authentication , where the user can select their passkey profile to sign-in with. |
options | LIDOptions? | No | Additional options for the sign-in process. |
options.usernameType | String | No | Specify username type validation. Types include email or phone . |
onComplete | Closure: (LIDAuthResponse?, LIDApiError?) -> Void | Yes | A completion handler that is called when the authentication process completes, returning either an LIDAuthResponse or an LIDApiError . |
generateCodeWithPasskey
Generates a code by authenticating with a passkey for the specified username and purpose. The response will include an OTP that can be used for other forms of authentication and recovery flows.
Have a look at the recovery flow guide on how to implement this feature.
public func generateCodeWithPasskey(
anchor: ASPresentationAnchor,
username: String,
options: LIDOptions?,
onComplete: @escaping(LIDCodeResponse?, LIDApiError?) -> Void
)
Parameter | Type | Required | Details |
---|---|---|---|
anchor | ASPresentationAnchor | Yes | A presentation anchor, typically a window or a view controller, that the authentication UI should be presented from. |
username | String | Yes | The username for the account attempting to authenticate. Passing an empty string will trigger usernameless authentication , where the user can select their passkey profile to sign-in with. |
options | LIDOptions? | No | Additional options for the sign-in process. |
options.usernameType | String | No | Specify username type validation. Types include email or phone . |
onComplete | Closure: (LIDCodeResponse?, LIDApiError?) -> Void | Yes | A completion handler that is called when the authentication process completes, returning either an LIDCodeResponse or an LIDApiError . |
sendCode
Sends an authentication code to the specified user via the chosen method. The username must have either an email
or phone
profile for the method to work.
Use the authenticateWithCode method to verify the code sent to the user.
For further implementation details have a look at the cross device authentication guide.
@objc public func sendCode(
username: String,
method: String = "email",
options: LIDOptions?,
onComplete: @escaping(LIDApiError?) -> Void
)
Parameter | Type | Required | Details |
---|---|---|---|
username | String | Yes | The username for the account to which the code will be sent. |
method | String | No | The method to use for sending the code. Valid values are email (default) and sms . |
options | LIDOptions? | No | Additional options for the request. |
options.usernameType | String | No | Specify username type validation. Types include email or phone . |
onComplete | Closure | Yes | A completion handler that is called when the confirmation authentication process completes, returning an LIDApiError or nil . |
authenticateWithCode
Authenticate with one-time authentication for the given username.
Have a look at the recovery flow guide on how to implement this feature.
public func authenticateWithCode(
username: String,
code: String,
options: LIDOptions?,
onComplete: @escaping(LIDAuthResponse?, LIDApiError?) -> Void
)
Parameter | Type | Required | Details |
---|---|---|---|
username | String | Yes | The username for the account attempting to authenticate. |
code | String | Yes | The OTP code to authenticate. |
options | LIDOptions? | No | Additional options for the sign-in process. |
options.usernameType | String | No | Specify username type validation. Types include email or phone . |
onComplete | Closure: (LIDAuthResponse?, LIDApiError?) -> Void | Yes | A completion handler that is called when the authentication process completes, returning either an LIDAuthResponse or an LIDApiError . |
confirmTransaction
Enables secure transaction confirmation using a passkey, incorporating transaction validation for actions such as payments or modifications to sensitive account information. This method ensures that the transaction is authorized by the rightful passkey owner. In all, it is similar to authenticateWithPasskey with extra data related to the transaction.
Have a look at the transaction confirmation guide on how to use this.
public func confirmTransaction(
anchor: ASPresentationAnchor,
username: String,
txPayload: String,
options: LIDOptions?,
onComplete: @escaping (LIDAuthResponse?, LIDApiError?) -> Void
)
Parameter | Type | Required | Details |
---|---|---|---|
anchor | ASPresentationAnchor | Yes | A presentation anchor, typically a window or a view controller, that the transaction confirmation UI should be presented from. |
username | String | Yes | The username for the account attempting to perform transaction confirmation. |
txPayload | String | Yes | The transaction-specific payload, containing details like transaction amount, recipient, and other necessary metadata. |
options | LIDOptions? | No | Additional options for transaction confirmation, including transaction type and other relevant information. |
options.nonce | String | No | A unique nonce to ensure the transaction's integrity and prevent replay attacks |
options.txType | String | No | Specify the type of transaction being confirmed for additional validation. |
onComplete | Closure: (LIDAuthResponse?, LIDApiError?) -> Void | Yes | A completion handler that is called when the confirmation authentication process completes, returning either an LIDAuthResponse or an LIDApiError . |
listPasskeys
Retrieve a list of the users' passkeys from the server.
Requires one of the following:
- User must be signed in.
- Usage of authorization tokens.
public func listPasskeys(
options: LIDOptions?,
onComplete: @escaping ([LIDPasskeyInfo]?, LIDApiError?) -> Void
)
Parameter | Type | Required | Details |
---|---|---|---|
options | LIDOptions? | No | Additional options for the list passkeys process. |
options.token | String | No | The user's authentication token, which may also be a management token with the scope passkey:read . |
onComplete | Closure: ([LIDPasskeyInfo]?, LIDApiError?) -> Void | Yes | A completion handler that is called when the confirmation authentication process completes, returning either an [LIDPasskeyInfo] or an LIDApiError . |
renamePasskey
Renames a passkey user credential.
Requires one of the following:
- User must be signed in.
- Usage of authorization tokens.
public func renamePasskey(
passkeyId: String,
name: String,
options: LIDOptions?,
onComplete: @escaping (LIDApiError?) -> Void
)
Parameter | Type | Required | Details |
---|---|---|---|
passkeyId | String | Yes | The UUID of the passkey attempting to rename. |
name | String | Yes | The new name for the passkey. |
options | LIDOptions? | No | Additional options for the renaming passkey process. |
options.token | string | No | The user's authentication token, which may also be a management token with the scope passkey:write . |
onComplete | Closure: (LIDApiError?) -> Void | Yes | A completion handler that is called when the confirmation authentication process completes, returning an LIDApiError or nil . |
deletePasskey
Deletes a passkey user credential.
Requires one of the following:
- User must be signed in.
- Usage of authorization tokens.
public func deletePasskey(
passkeyId: String,
options: LIDOptions?,
onComplete: @escaping (LIDApiError?) -> Void
)
Parameter | Type | Required | Details |
---|---|---|---|
passkeyId | String | Yes | The UUID of the passkey attempting to delete. |
options | LIDOptions? | No | Additional options for the delete passkey process. |
options.token | string | No | The user's authentication token, which may also be a management token with the scope passkey:write . |
onComplete | Closure: (LIDApiError?) -> Void | Yes | A completion handler that is called when the confirmation authentication process completes, returning an LIDApiError or nil . |
isLoggedIn
Check if a given user is currently logged in.
None.
public func isLoggedIn() -> Bool
getUser
Retrieves the authenticated user data.
public func getUser() -> LIDUser
signout
Signs out the authenticated user, if the user is set.
None.
public func signout() -> Void
The iOS SDK does not automatically remember if a user is logged in once the app is closed. Functions like isLoggedIn, getUser, and signout work only while the app remains open. Developers, for now, will need to manually manage user sessions to maintain login status across app restarts.
Errors
LIDApiError
Can occur during the authentication process. It is designed to encapsulate detailed information about login-related errors, making it easier to handle and debug issues related to user authentication.
Field | Type | Details |
---|---|---|
errorCode | string | The error code associated with the login error. |
errorMessage | string | The detailed message or description of the error. |